Iced IoT
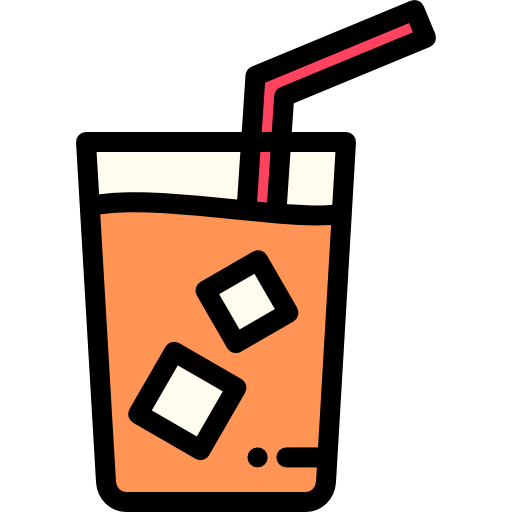
Table of Contents
Features
- RealTime Device Information: Accurate and up to date info and analytics
- Scalable: Built with Docker, CosmosDB, and NGINX for scalability and resilience
- Modular: Microservices architecture
- Current: Built with modern cloud tooling and design patterns
Design Philosophy
Iced IoT is a low overhead and robust IoT solution. The user can add facilities and devices of any variety to the IoT hub with minimal configuration. Added devices are instantly visible on the web app for tracking and analytics.
The platform is a series of microservices that can be independently scaled and maintained. The entire platform is written in JavaScript with heavy use of non-blocking async code. The React front end is an MVC-style app employing the presentation/container pattern. All application services are dockerized and running as container instances in Azure. Continuous integration and delivery are handled by CircleCI.
Platform Architecture
Associated Repo's
- Express API's
- Backend structure for the React frontent
- Azure Function App
- Re-directing IoT hub messages into CosmosDB
- IoT Devices
- Simulated IoT devices and facilities
Tools
- React
- Frontend Framework
- NGINX
- Webserver
- Docker
- Container platform
- Azure IoT Hub
- Two way IoT device communication
- Azure Functions
- Serverless compute
- CosmosDB
- Globally distributed, multi-model database service
- Node.js
- JavaScript runtime
- Express.js
- Node.js web application framework
- CircleCI
- Continuous integration and delivery service
iot-sim 
Representational simulation of IoT devices.
Table of Contents
About
This project creates simulated IoT devices and facilities. Realistic data is generated based on the type of device and time of day. IoT telemetry data can be handled by a custom user-defined function.
Devices
- Energy Device
- Tank Device
- Wind Device
Device API
/** * Device * * @constructor * @param {String} dsn - device serial number. * @param {String} type - type of device. * @param {String} connectionString - connection parameter for Azure IoT hub. * @param {String} profile - device signature. * @param {boolean} log - True=print fancy output console/False=no output * @param {function} handler - User defined function to handle the telemetry data */
Facility API
/**
* Facility
*
* Models the composition of IoT objects into a single IoT "Facility"
*
* @constructor
* @param {string} name - name of the facility.
* @param {array} devices - IoT devices in the facility.
* @param {array} geolocation - lat and lon of the facility.
* @param {number} frequency - milliseconds between messages.
*/
Usage
import {TankDevice, WindDevice, EnergyDevice, Facility} from "iot-sim"
// Facility 1
// Tank Monitoring Device
const tank_iot_01 = new TankDevice({
dsn: "tank_iot_01",
type: "tank",
profile: null,
log: false,
handler: console.log
});
// Wind Monitoring Device
const wind_iot_01 = new WindDevice({
dsn: "wind_iot_01",
type: "wind",
profile: "profile1",
log: false,
handler: console.log
});
// Energy Monitoring Device
const energy_iot_01 = new EnergyDevice({
dsn: "energy_iot_01",
type: "energy",
profile: "profile1",
log: false,
handler: console.log
});
const facility_01 = new Facility({
name: "facility_01",
devices: [energy_iot_01, wind_iot_01, tank_iot_01],
geolocation: {
lat: 51.0447,
lon: 114.0719
},
frequency: 10000,
});
facility_01.setupFacility();
facility_01.putOnline();
Output data
{ data: { watts: 171.189 }, timestamp: 1586550588454, id: 'a855447a-b488-475b-bb2c-be80cabba8ca', _id: 'a855447a-b488-475b-bb2c-be80cabba8ca', dsn: 'energy_iot_01', geolocation: { lat: 51.0447, lon: 114.0719 }, facility: 'facility_01', type: 'energy' } { data: { mph: 20.995, direction: 'S' }, timestamp: 1586550588459, id: '6042d595-b93f-4ff8-884a-38d860fa73c4', _id: '6042d595-b93f-4ff8-884a-38d860fa73c4', dsn: 'wind_iot_01', geolocation: { lat: 51.0447, lon: 114.0719 }, facility: 'facility_01', type: 'wind' } { data: { liters: 98.404, pH: 6.079 }, timestamp: 1586550588460, id: 'd2befdfa-b2d9-4b9a-8970-523c486346b1', _id: 'd2befdfa-b2d9-4b9a-8970-523c486346b1', dsn: 'tank_iot_01', geolocation: { lat: 51.0447, lon: 114.0719 }, facility: 'facility_01', type: 'tank' }
iotsimbackend
REST API for simulated IoT device data
iotsimbackend
is a simple express API server built on a MongoDB database. This repo serves as the backend for the Iced Iot React.js dashboard application.Table of Contents
Available Scripts
In the project directory, you can run:
npm start
npm start
Runs the app in production mode. Open http://localhost:3005 to view data in the browser.
npm start:dev
npm start:dev
Runs the app with hot module reloading
npm docker
npm docker
Script called by Dockerfile for build
Endpoint
Endpoint | Request Type | Description |
---|---|---|
{host}/api/devices/ | GET | Returns all device data points |
URL Paramaters
These parameters can be used in combination with each other
Parameter | Request Type | Description |
---|---|---|
/facility/
| GET | Returns all device data points in a facility |
/dsn/
| GET | Returns all device data points for a device serial number |
/type/
| GET | Returns all device data points for an IoT device type |
/gte/
| GET | Returns all device data points after specified date |
Deployment
This codebase can be deployed as a container via docker-compose
docker-compose build docker-compose up
Open http://localhost:3005 to view data in the browser.
Built With
IoTHub_EventHub_MongoDB
Send Azure IoT hub messages to CosmosDB (MongoDB)
About
Azure Function App for routing IoT hub messages to an external MongoDB database.
Built With
- Node.js
- JavaScript runtime